In this tutorial, we are going to create pagination with PHP and MySql. It is probably possible that your SQL SELECT query may return results into thousand and millions of records. And it is obviously not a good idea to display all those results on a single page. So we can split this result into multiple pages.
What is Pagination?
Paging means displaying all your fetched results in multiple pages instead of showing them all on one page. It makes that page so long and would take so much time to load.
How to create Pagination with PHP and MySql
MySQL’s LIMIT clause helps us to create a pagination feature. It uses two arguments First argument as OFFSET
and the second argument the number of records which will be returned from the database.
You can find ajax version for this tutorial in my other article ajax pagination here.
Follow these simple steps to create pagination in PHP –
1. Get the current page number
This code will get the current page number with the help of $_GET
Array. Note that if it is not present it will set the default page number to 1.
if (isset($_GET['pageno'])) {
$pageno = $_GET['pageno'];
} else {
$pageno = 1;
}
2. The formula for php pagination
You can always manage the number of records to be displayed in a page by changing the value of $no_of_records_per_page
variable.
$no_of_records_per_page = 10;
$offset = ($pageno-1) * $no_of_records_per_page;
3. Get the number of total number of pages
$total_pages_sql = "SELECT COUNT(*) FROM table";
$result = mysqli_query($conn,$total_pages_sql);
$total_rows = mysqli_fetch_array($result)[0];
$total_pages = ceil($total_rows / $no_of_records_per_page);
4. Constructing the SQL Query for pagination
$sql = "SELECT * FROM table LIMIT $offset, $no_of_records_per_page";
5. Pagination buttons
These Buttons are served to users as Next Page
& Previous Page
, so that they can easily navigate through you pages. Here I am using bootstrap’s pagination button, you can use your own buttons if you want.
<ul class="pagination">
<li><a href="?pageno=1">First</a></li>
<li class="<?php if($pageno <= 1){ echo 'disabled'; } ?>">
<a href="<?php if($pageno <= 1){ echo '#'; } else { echo "?pageno=".($pageno - 1); } ?>">Prev</a>
</li>
<li class="<?php if($pageno >= $total_pages){ echo 'disabled'; } ?>">
<a href="<?php if($pageno >= $total_pages){ echo '#'; } else { echo "?pageno=".($pageno + 1); } ?>">Next</a>
</li>
<li><a href="?pageno=<?php echo $total_pages; ?>">Last</a></li>
</ul>
6. Let’s assemble all the codes in one page
<html>
<head>
<title>Pagination</title>
<!-- Bootstrap CDN -->
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css">
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.2.1/jquery.min.js"></script>
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/js/bootstrap.min.js"></script>
</head>
<body>
<?php
if (isset($_GET['pageno'])) {
$pageno = $_GET['pageno'];
} else {
$pageno = 1;
}
$no_of_records_per_page = 10;
$offset = ($pageno-1) * $no_of_records_per_page;
$conn=mysqli_connect("localhost","my_user","my_password","my_db");
// Check connection
if (mysqli_connect_errno()){
echo "Failed to connect to MySQL: " . mysqli_connect_error();
die();
}
$total_pages_sql = "SELECT COUNT(*) FROM table";
$result = mysqli_query($conn,$total_pages_sql);
$total_rows = mysqli_fetch_array($result)[0];
$total_pages = ceil($total_rows / $no_of_records_per_page);
$sql = "SELECT * FROM table LIMIT $offset, $no_of_records_per_page";
$res_data = mysqli_query($conn,$sql);
while($row = mysqli_fetch_array($res_data)){
//here goes the data
}
mysqli_close($conn);
?>
<ul class="pagination">
<li><a href="?pageno=1">First</a></li>
<li class="<?php if($pageno <= 1){ echo 'disabled'; } ?>">
<a href="<?php if($pageno <= 1){ echo '#'; } else { echo "?pageno=".($pageno - 1); } ?>">Prev</a>
</li>
<li class="<?php if($pageno >= $total_pages){ echo 'disabled'; } ?>">
<a href="<?php if($pageno >= $total_pages){ echo '#'; } else { echo "?pageno=".($pageno + 1); } ?>">Next</a>
</li>
<li><a href="?pageno=<?php echo $total_pages; ?>">Last</a></li>
</ul>
</body>
</html>
That’s all!
If you find this article helpful, don’t forget to share it with your friends. And stay updated with us by subscribing our blog.
Please help us by showing your reaction
very nice way to describe things…keep doing the good work
Thank you for your valuable feedback @Rohit.
nice article tutor sir, i will try it on my code. thanks.
hi Paritosh
dude you doing the best job for the beginners who start a career in web development
Hello @Jass,
Thank you for appreciating and keep visiting.
Regards
Hey keep posting such good and meaningful articles.
When I use a submit form with post and the query with select * from xxx Like “search”, when I click to another page the post variable is gone. How to fix this?
you just have to pass your searched variable in pagination buttons (in URI GET param),
append this in pagination buttons
&q=$searchedVariable
So i’m trying to do what you said and its not going well.
what is exactly the searched variable is it the $query=select * from xxx Like “search”? or something else?
Hello! I’m to stupid to check this 🙂
where should i add this?
// Check connection
if (mysqli_connect_errno()){
echo “Failed to connect to MySQL: ” . mysqli_connect_error();
die();
}
$total_pages_sql = “SELECT COUNT(*) FROM customers”;
$result = mysqli_query($conn,$total_pages_sql);
$total_rows = mysqli_fetch_array($result)[0];
$total_pages = ceil($total_rows / $no_of_records_per_page);
$sql = “SELECT * FROM customers LIMIT $offset, $no_of_records_per_page”;
$search = isset($_POST[‘search’]) ? $_POST[‘search’] : ”;
$sql = “SELECT * FROM customers WHERE NAME1 LIKE ‘%$search%’ OR STREET LIKE ‘%$search%’ LIMIT $offset, $no_of_records_per_page”;
$res_data = mysqli_query($conn,$sql);
while($row = mysqli_fetch_array($res_data)){
echo “”;
echo “” . $row[‘ID’] . “”;
echo “” . $row[‘NAME’] . “”;
echo “” . $row[‘STREET’] . “”;
echo “”;
echo ““;
echo ““;
echo ““;
echo “”;
echo “”;
}
echo “”;
mysqli_close($conn);
?>
First
<li class="<?php if($pageno “>
<a href="<?php if($pageno “>Prev
<li class="= $total_pages){ echo ‘disabled’; } ?>”>
<a href="= $total_pages){ echo ‘#’; } else { echo “?pageno=”.($pageno + 1); } ?>”>Next
<a href="?pageno=”>Last
l;;p
thanks bro this is useful for me
Me too facing this challenge. Can you help about this?
am getting an error of undefined variable
pegno of get array
double check the code, it is not
$pegno
actually it is
$pageno
Great Tutorial! This PHP pagination tutorial have all the options. But I think it would be better if you add an option to change the total number of results. What do you think about it?
Hello @Souradeep,
Thank you for such valuable comments. And yeah its a good idea, I definitely gonna implement your suggestion.
nice tutorial but i have some problem i am gettng error
undefined pageno
Hello @Edrine,
please make sure to place below code at the top of page
Hey,
Great tutorial mate.
But I’ve got some GET variables in the URL, which are lost when I press the NEXT or any other button. It just edits the URL to “?pageno=2”.
It’d great if you fixed that. Thanks!
Hello Ali,
all you have to do is pass your GET parameters in pagination buttons too.
Hey Paritosh,
Thanks for your reply. How do I do that? Any pointer?
Thanks again!
suppose you have 2 get params (type & status) in your url
then pagination code would be
Bro, you did a great job..
Really appreciated,
Thanks
Thank you for appreciating Mr. Hamza, keep visiting.
Good day. The paginator is displaying at the bottom but when I click next, it returns empty results.
could it be possible to provide a demo link for your paginator, it helps me to understand better.
Am getting an error that a non numeric value was encountered on the line holding the next page code am new to php help
try placing
(int)
keyword in front of$pageno
. Like this(int)$pageno
Nice tutorial overall, how do I re write the URL in to something like this
https://www.myprogrammingtutorials.com/page/2
? Any tip will be of great help
you can use
RewriteRule ^(.*)$ index.php?params=$1 [NC]
in your .htaccess file, so that your old urlhttp://url.com/index.php?params=value
be like
http://url.com/params/value
Hi Paritosh, I am trying to echo $result but I get this message: Resource id #17 when I do. Please what is the cause?
good posting good website
Thank you for your appreciating words @maliksanwal
Aaaaaa, thanks sir!! just now i got it xD
happy to hear ya! @scarlet
Hi, in the process of writing the code I try to echo $result before I proceed but got this message: Resource id #17. Please how do i sort it out?
please let us see the whole error if possible, it’ll help me to figure the problem out.
I didn’t get an error, I only got the message: Resource id #17. please I need help, I’ve been battling with this for day…
after echo the
$result
variable you should getObject of mysqli_result couldn’t be converted to string
error
dude when i clicked on next, its always launch me to other page, how can i fix this? thanks
please let me have a look your pagination buttons code.
Hi,
how do I get page numbers to show?
you could use
Very Informative.
Make a tutorial on nested comments in php
Thank you for appreciating @Faisal
sure I will post a tutorial on nested comments, meanwhile, you can use
“https://www.myprogrammingtutorials.com/multi-level-nested-category-system-codeigniter-mysql.html” concept for your nested comments.
Thank you for this tutorial, I encountered some problem
my url is already ?pageno=2 but the table is still at page 1.
it’d help me to understand better if you could provide me your app’s link.
I’m working on local server
make sure to place this snippet in right way
not displaying data
i don’t know if i should add the field of table
Thank
please let us see your php codes snippet
Thanks man. It was really helpful. Thank you very much
@Sahan glad it helped.
i dont understand the pageno meanining in the first isset line is it a functio or what and please describe about $offset = ($pageno-1) * $no_of_records_per_page;
The
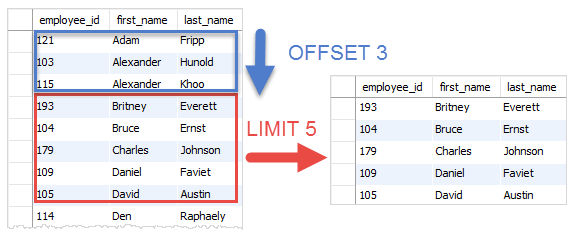
OFFSET
clause skips theoffset
rows before beginning to return the rows. Maybe this image can help you understand betterwhile($row = mysqli_fetch_array($res_data)){
//here goes the data
}
What data goes in while loop?????
your actual data what you’re paginating at, it could be in
<table>
or in any element you wantGood Job, really appreciate the script…been having a hard time implementing serial numbering that increments on every page
The Code is working great
any possibility to put the pagination buttons on top of the table ?
when i do, they don’t function
it’s really helpful thanks a lot.
glad!!!
i want to know how to show the result of search query using pagination
Sir,
THANKS A LOT
Very good work
thanks
Thank you so much!
Hey,
I am using exactly the same code as yours, but not getting the desired result.
I am not sure how to modify the href according to my web page. Can you please check my code and see if you can help me modify it and make it work?
please let us see your code
Thank you
https://stackoverflow.com/questions/52839830/add-pagination-to-list-grid-view-in-php-bootstrap-css/52936228?noredirect=1#comment92809409_52936228
You should use AJAX pagination here, loading both views (List & Grid) is not a good practice, let me show you pointwise flow
– serve view buttons (List & Grid) initially and make one default view
– put “Next Button” downside
– when a user clicks on “Next Button”, find his/her selected view (List & Grid) and make an AJAX request along with the selected view value
– according to the view value, you can return its relevant html codes.
– at last append response into the final container
here is a good tutorial about ajax pagination
Sir,
THANKS A LOT
Very good work
thanks
glad it helped, and thank you for appreciating BTW
This was really simple, great tutorial.
But, why my page not show the number?
Just :
FirstPrevNextLast
Thanks before.
I try to use with nested loop, but iam stuck, could you help me?
This is my query :
query($query);
$total_records = $result->num_rows;
while($row = $result->fetch_array()) {
$datarows[] = $row;
}
$num_cols = 3;
$num_rows = ceil($total_records / $num_cols);
$num = 0;
echo “\n”;
// next the loop for the table rows
for ($rows = 0; $rows < $num_rows; $rows++) { echo "\n”; // this is the loop for the table columns for ($cols = 0; $cols < $num_cols; $cols++) { if ($num < $total_records) { // first create variables with the values of the current record $1 = $datarows[$num]['1']; $2 = $datarows[$num]['2']; $3 = $datarows[$num]['3']; echo " “.$1.” – “.$2.” – “.$3.” \n”; } else { // show an empty cell echo ” \n”; } $num++; } echo “\n”; } echo “\n”; ?>
Nice tutorial but I believe your pagination example can be improved by also disabling the First and Last pagination buttons if you are currently on one of those pages.
This is easily done so I won’t insult you by posting the code here! 🙂
its always good suggesting improvements, I’ll definitely gonna implement you suggested,
Regards,
How do I display/get the numbers between 0-10 instead of displaying prev and next button?
you could use
$total_pages
in for loopThanks it’s helpfull. Nice blog 🙂
Hello and thx for this amazing tutoriel,
I have a problem with this system, might be from my own code, could you help me?
The pagination’s buttons working good, but the list limit isnt working.
Could you help me in private mb?
Thx a lot and nice job man, that’s amazing.
HEY BRO CHECK YOUR QUEEY THEN PUT IN THE LIMIT AND CHECK
THIS IS THE SYNTAX
($OW=MYSQLI_QYUEEY(“FROM TABEL LIMIT 10, 20 “))
i want to show some number of pages as well
hi there i use this code for my listing page but i got error which icludes this message…
Message: mysqli_fetch_array() expects parameter 1 to be mysqli_result, boolean given
hello
i use your code for my search page but i have 2 error’s include this message :-
mysqli_fetch_array() expects parameter 1 to be mysqli_result, null given in ..
mysqli_query() expects parameter 1 to be mysqli, object given in …
please help me , and thank you .
I’m new in PHP. And this article is so much helpfull people like me from beginning class. Thank you so much my firend! You did wonderfull job!
Always the first line on the page is missing?
$no_of_records_per_page = 5; and there is 14 rows total.
The first page is 2…5
The next page is 7…10
The last page is 12…14.
Excellent tutorial, shown from beginning to end, the way it should be.
this is what I was looking for.
thank you for your hard working.
Thank you so much, this solved my problem?
Two questions?
How do I make pagination for image galleries?
How do I give a dynamic background color for active elements of pagination?
Thank you
you could use
$total_pages
in for loopyou could use above strategy in your pagination buttons
Thanks so much, this really helped me. I have searched all around for pagination like this, I really appreciate.
Glad it helped!, Thank you.
Thanks, You are a Genius. God bless you!
So far, I agree with you on much of the info you have written here. I will have to think some on it, but overall this is a wonderful article.
Thank you so much for sharing this pagination, very clear and easy to understand.
God bless you, brother!
Thank you for your feedback, keep visiting.
I paste that code in blog page in my blog page there are 3 content Image Title and Text but i pasted that code and change the number of page record means i want only 3 blog per page and go to next page but that code not work please reply my question as soon as fast
That was useful code that I was looking for. I have added another column to the table to give it an edit capability with Edit and it works fine with an edit screen and n is dynamically generated.
Thank you Paritosh ji for sharing this code which saved me a lot of time.
mysqli_fetch_array() expects parameter 1 to be mysqli_result, bool given in line 52 where i have this code
while($row = mysqli_fetch_array($query))
{
print_r($row);
}
Can you help me please?
thank you this helped me alot
Glad it helped ya, keep visiting.
while($row = mysqli_fetch_array($query))
{
$obj[] = $row;
}
foreach ($obj as $user){
echo ”.$user[“username”].”;
}
Tutoriel Simple et efficace, l’essentiel pour avoir une base solide. Puis chercher à l’améliorer. Merci pour cette aide, e me noyait dans les tutoriaux tellement nombreux sur ce sujet.
(google translation)
Tutorial Simple and effective, the essential to have a solid foundation. Then try to improve it. Thank you for this help, I was drowning in so many tutorials on this topic.
Best reguards
Marc
Great tutorial.
How to show all pages instead of First,Previous,Next and Last?
Example: I have 100 data to display which I would limit it to 10 per pages.
I want to direct click on page 8 instead of keep clicking on Next button.
Any guidelines? Thanks
Untested, but something like:
while ($x ‘.$pageno.’‘;
}
}
great tutorial, very usefull and solve my problem
Thank you very much for this. Not only was the code useful but the step-by-step made it very easy to adapt
Perfect!! just what I needed, Simple and easy
glad it helped.
Thank you very much I was searching for the sql query I was lost in it and you really helped me.
Thank You So Much Again!!
I understood the logic
Bless you! blended well with my script
A good tutorial for learning. Thanks
Thanks alot. Using your insturction i could simply insert the pagination to my data.
I have another question:
There are only First, Previous, Next and Last buttons.
Is it possible to add the nummber buttons for pages besid these buttons. As the “pagination” of Google?
good Tutorial for learning thanks
love u man thank u very much for this tuto .
I am getting an error i like this,
mysql_fetch_array() expects parameter 1 to be resource, boolean given in…..
I can’t use mysqli,because,in my project am using the earlier version.
Here is my code:
$link = mysql_connect($Host, $User, $Password) or die(‘Could not connect: ‘ . mysql_error());
mysql_select_db($DBName) or die(‘Could not select database’);
$total_pages_sql=”SELECT * from table”;
$result = mysql_query($total_pages_sql);
$total_rows = mysql_fetch_array($result,MYSQLI_NUM)[0];
$total_pages = ceil($total_rows / $no_of_records_per_page);
you can modify codes as per mysql needs
sir i have created a blog page. and it is working properly but 1 and 2 page post properly show . but when i click next page(3) it is showing blank and other page (like 4,5,6….) also.. i can not find where is my error.please help me why it can not show after 2 page..
I need to check the code snippets to figure it out.
ok i will send you code ..please sir give your mail id
[email protected]
sir i have sent it your mail id … please check it
sir please reply me …what is the status my issue
I didn’t received your mail.
Thank you
Thank for your post, but my dream viewer said has error in ” $total_rows = mysqli_fetch_array($result)[0];” how can i solve please….
Nice tutorial. I got many references.
Superb Work Man!
Great Tutorial have included it with some minor modification so it displays 100% for what i need only issue i have is i have records that shows a visit count per member and when i adjust the $sql to
$sql = “SELECT * FROM dxd_members LIMIT $offset, $no_of_records_per_page ORDER BY visits DESC”;
so that is lists members by the visit count highest to lowest i am getting this error
Warning: mysqli_fetch_array() expects parameter 1 to be mysqli_result, bool given in C:\Server\data\htdocs\dxdadmin\inc\member_visits.inc.php on line 61
How can i fix this so that i can have it shows my members by the number of visits highest to lowest.
Thanks
please post the codes atleast from line no 60 – 65 of your file “member_visits.inc.php” in order to identify the problem.
Thanks For This Post.
very nice i appriciate you thank you so much bro
Hello Paritosh, Nice tutorial. I am trying to implement this code in my project but whenever i click next page it returns empty. Cn you please take a look at my code and help me out.
https://imgur.com/a/XmDk1L7
as I can see here (at line no 100), you’re using
$result
variable instead of$result1
.There are two variables result, result1. i am doing this for result.
though it is hard to diagnose like this, my suggestion would be
#try diagnosing step by step, like whether or not any queries are getting executed and returning any data?
#if it returns, move ahead to next query.
#see error logs
#use exception.
it worked amazing for me bah how can i ignore my first 5 posts before i limit the number of post to show
like this
$query = “SELECT * FROM article a INNER JOIN
article_category ac
WHERE a.article_cat_id=ac.article_cat_id
AND ac.article_category=’General News’
ORDER BY posted_time DESC
LIMIT 5, 4”;
here the code will ignore the first 5 and limit to show 4
how do i do that to yours codes
thank u
you need to do some hard code here, first check whether it is first page or not, if it is a first page the set the
$offset
to 5(hardcoded). like soFirst of all, thank you. I have 10 yields. I want to divide it into 2 pages. It creates 2 pages. There is no problem here. It shows the same 10 data on 2 pages.
Hello ibrahim,
it is hard to find the problem this way, if it could be possible to share your codes, please do so.
Really helpful.
It worked perfectly for me!
Thanks
It is good but it does not work for me 🙂 I am asking the source of the problem.
It’s very helpful dear for my website. Thanks and keep it up.
Hello , the code is not full , where is sql? I cant use it in practise.
you just put some buttons , thats all? it gives error and I dont see anything except of buttons, why you did it , why you confuse all people, why its so bad.
I cant understand what I NEED myself here, why you didnt full it with pages and
with html and sql codes. You just wrote some php code with that is impossible to do something
please refer to point no. 6,
It’s very helpful dear for my website. Thanks and keep it up.
hello, please itried implementing it on a search filter but didnt work… please help…
it isn’t possible to find out what exactly going wrong in your codes.
please submit codes at MPT Community https://help.myprogrammingtutorials.com
Thank you
is it possible to do it in simple way
this is simple…
Hey,
I’m using this code, but I have a problem loading on diffrent pages.
In my index page I’m using this code
So in my main page it’s working fine where I listed some stuff, but in admin page where I added button “Edit” it’s only showing on first page, and when I’m loading pageno=2, it’s showing me the result from my main page, without button.
It works fine if I put it in main page.
Please Help!
Sorry, don’t know where code disappeared
I need pagination with bootstrap 3 columns. can you help me, please?
bhai kasam se dil se sukriya yara kal se itne plugins laga kr dekh chuka hu itni sari codings dekhi but bhai bht light tarike se apne bht accha explain karte huye mast sikha diya no need of any plugin now thnks a lot bhai
You can improve this by printing the previous, current and next page numbers in numeric digit form in the middle of the first/prev/next/last buttons.
I made the following code:
1){echo ($pageno – 1).” “;} echo ““.$pageno.” “; if($pageno
well, the comment system stripped my code out… but oh well
I really appreciate it @Dave, in my next update I definitely will implement these buttons.
Thank you
Your method of describing is awesome, thanks for sharing with us.
This Code is Codeigniter Api Create in pagination in error is every to page number change in show last recode this error how can sold
==============================================================================
public function product_data($category_id = null,$subcategory_id = null,$user_id = null,$page = 1)
{
if (!is_null($this->db))
{
/*$row = 10;
$row_pre = ($page * $row) – $row;*/
$this->db->select(‘*’);
$this->db->from(“products”);
if(!is_null($category_id) || !is_null($subcategory_id)){
$this->db->where(‘category_id’, $category_id);
$this->db->where(‘subcategory_id’, $subcategory_id);
}
$query_o = $this->db->get();
$result_o=$query_o->result();
$total_rows = COUNT($result_o);
$row_per_page = 10;
$begin = ($page – 1) * $row_per_page;
/*print_r($begin);
echo “”;*/
$total_pages = ceil($total_rows / $row_per_page);
/*print_r($total_pages);
exit;*/
if($page db->select(‘subcategory.name_sub as subcategory ,category.name as category,p.*,(SELECT qty FROM `carts` where prodect_id = p.id AND user_id = ‘.$user_id.’ ) as qty_sol,(SELECT id FROM `carts` where prodect_id = p.id AND user_id = ‘.$user_id.’ ) as carts_id,(SELECT is_favorite FROM `favorite` where prodect_id = p.id AND user_id = ‘.$user_id.’ ) as is_favorite’);
$this->db->from(“products p”);
$this->db->join(‘category’, ‘category.id = p.category_id’);
$this->db->join(‘subcategory’, ‘subcategory.id = p.subcategory_id’);
if(!is_null($category_id) || !is_null($subcategory_id)){
$this->db->where(‘p.category_id’, $category_id);
$this->db->where(‘p.subcategory_id’, $subcategory_id);
}
if (!is_null($page))
{
$this->db->limit($begin,$row_per_page);
//$this->db->order_by(‘p.id’, ‘ASC’);
}
$query_p = $this->db->get();
echo $this->db->last_query();
exit;
$result_p=$query_p->result();
print_r($result_p);
exit;
$data = array();
if(!empty($result_p))
{
foreach ($result_p as $value_p)
{
$data[] = array(
‘id’=>$value_p->id,
‘subcategory’=>($value_p->subcategory == Null) ? ” : $value_p->subcategory,
‘category’=>$value_p->category,
‘name’=>$value_p->name,
‘amount’=>$value_p->amount,
‘amount_discount’=>$value_p->amount_discount,
‘description’=>$value_p->description,
‘cart_id’=>($value_p->carts_id == Null) ? ‘0’ : $value_p->carts_id,
‘qty’=>($value_p->qty_sol == Null) ? ‘0’ : $value_p->qty_sol,
‘is_favorite’=>($value_p->is_favorite == Null) ? ‘0’ : $value_p->is_favorite,
‘images’=>json_decode($value_p->images),
‘availability’=>$value_p->availability
);
}
return $data;
}
else
{
return false;
}
}
else
{
echo “string”;
return false;
}
}
}
Thanks Its Working For me…….
Thank you so much for this post….
Thank you very much for you tutorial. 11 hours googling, my backbone was hurting so much, but you ´ve save me!!!
hi,
its working when i use ($sql=”SELECT * FROM products”;).
how do i make it work when use filters like ($sql=”SELECT * FROM products WHERE town=’$town’ AND property_type=’$property_type’”;)?
thanks in advance
please give it a try, it’ll work with them too
It works with the 1st page only. Next page is blank.
could it be possible to share your codes? atleast the sql query.
hi sir, i shared the code but its not showing here!
please use https://help.myprogrammingtutorials.com
in regards to How to create Pagination with PHP and MySQL
you asked me to share my codes. when i post they don’t appear here
please use MPT Community to ask your questions and share your codes there
https://help.myprogrammingtutorials.com/
Hello , Thank you for good posting and good tutorial.
how can I edit code to show last inserted row in the first ?
Hello
i have added same code you have given here inside page but not working properly.
Good blog, Paritosh. The bolg is well written in steps and the entire process of creating pagination with PHP and MySQL is explained well. The blog gives a clear idea as to how to do pagination and the code is also given. The information will be useful for those learning.
That works just great, thank you, simple and effective !
im having a challege here cos the code below
$total_pages_sql = “SELECT COUNT(hid) FROM crystalbeds_hotel_info where hotel_region = ‘south_east'”;
is outputing data even when criteria not met. pls help
i was able to solve the problem..didnt include the where clause in the second query
$sql = “SELECT * FROM crystalbeds_hotel_info where hotel_region = ‘south_east’LIMIT $offset, $no_of_records_per_page
Very nice work! it’s working
I noticed that the script is clear to me . but when i want to use it in my database which works with wampserver my query stops after current page other pages are linked but contain no output
Is this a wel known problem and how do i solve it
Hi, overall all is good but It works with the 1st page only. when we click on Next page, it’s given blank page?
Wow.Thank you very much.Can you sir explain the”[0]” in “$total_rows = mysqli_fetch_array($result)[0];”. Could don find related explanation.
Wao!
I am happy I stumbled over this.
It did not require much thinking, I implemented it in a blog and it worked without bugs.
I am so happy.
query($sql);
if ($page == 0){$page = 1;}
$prev = $page – 1;
$next = $page + 1;
$lastpage = ceil($total_pages/$limit);
$LastPagem1 = $lastpage – 1;
$paginate = ”;
if($lastpage > 1)
{
// $paginate.= “$counter
$paginate .= “”;
// Previous
if ($page > 1){
$paginate.= “previous“;
}else{
$paginate.= “previous”; }
// Pages
if ($lastpage < 7 + ($stages * 2)) // Not enough pages to breaking it up
{
for ($counter = 1; $counter <= $lastpage; $counter++)
{
if ($counter == $page){
$paginate.= "$counter”;
}else{
$paginate.= “$counter“;}
// $paginate.= “$counter“;}
}
}
elseif($lastpage > 5 + ($stages * 2)) // Enough pages to hide a few?
{
// Beginning only hide later pages
if($page < 1 + ($stages * 2))
{
for ($counter = 1; $counter < 4 + ($stages * 2); $counter++)
{
if ($counter == $page){
$paginate.= "$counter”;
}else{
//$paginate.= “$counter“;
$paginate.= “$counter“;}
}
$paginate.= “…”;
$paginate.= “$LastPagem1“;
$paginate.= “$lastpage“;
}
// Middle hide some front and some back
elseif($lastpage – ($stages * 2) > $page && $page > ($stages * 2))
{
$paginate.= “1“;
$paginate.= “2“;
$paginate.= “…”;
for ($counter = $page – $stages; $counter <= $page + $stages; $counter++)
{
if ($counter == $page){
$paginate.= "$counter”;
}else{
$paginate.= “$counter“;}
}
$paginate.= “…”;
$paginate.= “$LastPagem1“;
$paginate.= “$lastpage“;
}
// End only hide early pages
else
{
$targetpage = “job_searchs.php?q=result&searchfor=advancesearchs”;
$paginate.= “1“;
$paginate.= “2“;
$paginate.= “…”;
for ($counter = $lastpage – (2 + ($stages * 2)); $counter <= $lastpage; $counter++)
{
if ($counter == $page){
$paginate.= "$counter”;
}else{
$paginate.= “$counter“;}
}
}
}
// Next
if ($page < $counter – 1){
$paginate.= "next“;
}else{
$paginate.= “next”;
}
$paginate.= “”;
}
$number_of_results = mysqli_num_rows($result);
if($number_of_results > 0) {
# code…
while($row = $result->fetch_assoc()) {
?>
account_balance
.
.
place
Salary-
<a href="view-jobs.php?id=” class=”part-full-time”>View More
<?php
}
}
}else
{
echo 'No result found!…..’;
}?>
keyboard_arrow_left
Thank you, my other pagingation is bad, i changed, i did it hard for converted sqlite code.
could it be possible to share your codes? atleast the sql query.
please tell me
Nice article. I’m using ColdFusion, but you clearly defined each step and I was able to quickly convert the flow. Thanks!
sir please solve first error in code
i am getting error in $total_rows and my data inside database is is not showing.
i used different code from another site and those code work!
Hi, Im using PHPSense code for pagination on my website. Currently I have shifted my PHP version from 5.6 to 7.3 and the code stop working. Can you please help me with that.
Thanks
Please i’m using your code but when i click on next button, it throws an error that my $_GET[‘santino’] is undefined. The url which when clicked, goes to the pagination page, contains ‘santino’ as the value and that’s the value i use to call the items from the database by using the WHERE clause pointing to ‘santino’. Can you please help me solve it?
its very useful to me thank you so much
Fabulous! Well said.
This was passed on to me by a colleague one notch up leadership from me. I know he has heard me question but this article really put it into perspective.
its very useful to me thank you so much inquiry visit
Hi, when you click on the nav buttons you’re redirected to the top of the page, how do I stop this?
Apart from this, works great! Thank you
contains ‘santino’ as the value and that’s the value i use to call the items from the database by using the WHERE clause pointing to ‘santino’. Can you please help me solve it?
Thanks you brother, Its very useful to complete my site project
I want to creat Table of content in my wordpress website using codeing
how can i create this please reply
I am also a blogger and I work on my blog UPYojana.net
Where I shrar UP Government Scheme Related article to Internet User and Readers.
Recently I write an article on UP Labour Registration Online
and i want to add table of content in this article by coading.
thank’s sooooo much
you ar so generous and really genius
is useful simple and essential
🙂
really understandable even for php noob like me, thanks
Sir you are very great i liked your article i like it
Nice Article Sir
Ahref free backlink checker tool
thank you, sir, it’s very useful.
Nice website You are sharing a very informative and great post.I always share it with my friends and relatives 👍
Thank you for the super amazing blog, also addding one thing that UI of your website is very interesting.
Great tutorial of Pagination with PHP and MySql.
Very informative website keep sharing boss 👍
i’m not a programmer.
can someone help me to insert the pagination script onto my existing script here?
DEPOSIT MANAGEMENT SYSTEM
VIEW DEPOSITORIES
BACK TO SEARCH FORM
BACK TO MENU
$value) {
if ($key != ‘action’) {
if ($value != ”) {
$sql .= ‘ and ‘ . $key . ‘ like “%’ . $value . ‘%” ‘;
$criteria .= $key . ‘=’ . $value . ‘, ‘;
}
}
}
}
if ($criteria != ”) {
$criteria = substr($criteria, 0, strlen($criteria) – 2);
echo ‘Search criteria: ‘ . $criteria . ”;
}
$qry = $db->query($sql);
if ($qry->num_rows > 0) {
?>
NO
NAME
NRIC
CURR BAL
DATA TYPE
DATA SUBTYPE
ACTION
fetch_assoc()) {
$i++;
?>
<a class="hyperlink" href="dep_mgmt_form.php?id=”>VIEW
DATA NOT FOUNDPlease try again with a new search criteria
BACK TO SEARCH FORM
BACK TO MENU
Very helpful information sir ji
nice post and good information
Brilliant!!
thank you so much sir
Nice Information Thanks a lot
nice post and good information
Finally, I found the step-by-step instructions to be incredibly clear and easy to follow. The examples used in the tutorial were practical and relatable, making it easy for me to apply the concepts to my own projects.
I especially appreciated the attention to detail and the explanations provided for each step. The tutorial covered everything from setting up the database to creating the pagination links, which made it a comprehensive resource for anyone looking to implement pagination on their website.
Thankz 🙂
Great share! .Keep posting more like this!
Thanks a lot . Very helpful
Nice Post
Nice information sir
Thanks for sharing!